- Published on
Mastering Python Data Structures A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
Python is a powerful and versatile programming language that provides a range of data structures to store and manipulate data. Data structures are the building blocks of any programming language, and understanding them is crucial to write efficient and effective code. In this blog post, we will explore the different data structures in Python, including lists, tuples, dictionaries, and sets, and how to use them in your programming journey.
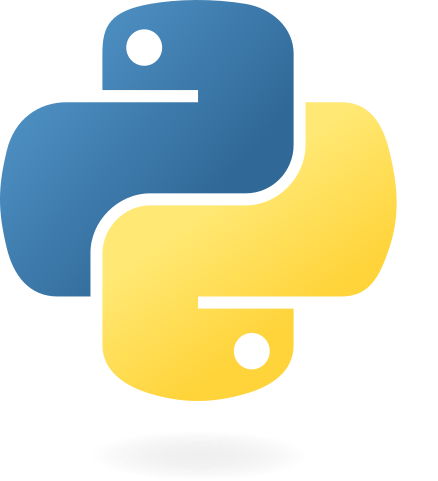
Lists
Lists are one of the most commonly used data structures in Python. A list is a collection of items that can be of any data type, including strings, integers, floats, and other lists. Lists are denoted by square brackets []
and are mutable, meaning they can be modified after creation.
Creating a List
To create a list, you can use the list()
function or simply enclose the elements in square brackets:
my_list = list([1, 2, 3, 4, 5])
or
my_list = [1, 2, 3, 4, 5]
Indexing and Slicing
List elements can be accessed using indexing, where the index starts from 0. You can also use slicing to extract a subset of elements from the list:
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Output: 1
print(my_list[1:3]) # Output: [2, 3]
List Operations
Lists support various operations, including:
- Append: adds an element to the end of the list
- Insert: inserts an element at a specific position in the list
- Extend: adds multiple elements to the end of the list
- Remove: removes the first occurrence of an element in the list
- Sort: sorts the list in ascending or descending order
- Reverse: reverses the order of the list
my_list = [1, 2, 3, 4, 5]
my_list.append(6) # Output: [1, 2, 3, 4, 5, 6]
my_list.insert(2, 7) # Output: [1, 2, 7, 3, 4, 5, 6]
my_list.extend([8, 9, 10]) # Output: [1, 2, 7, 3, 4, 5, 6, 8, 9, 10]
my_list.remove(7) # Output: [1, 2, 3, 4, 5, 6, 8, 9, 10]
my_list.sort() # Output: [1, 2, 3, 4, 5, 6, 8, 9, 10]
my_list.reverse() # Output: [10, 9, 8, 6, 5, 4, 3, 2, 1]
Tuples
Tuples are similar to lists, but they are immutable, meaning they cannot be modified after creation. Tuples are denoted by parentheses ()
and are often used when you need to store a collection of data that should not be changed.
Creating a Tuple
To create a tuple, you can use the tuple()
function or simply enclose the elements in parentheses:
my_tuple = tuple((1, 2, 3, 4, 5))
or
my_tuple = (1, 2, 3, 4, 5)
Indexing and Slicing
Tuple elements can be accessed using indexing, where the index starts from 0. You can also use slicing to extract a subset of elements from the tuple:
my_tuple = (1, 2, 3, 4, 5)
print(my_tuple[0]) # Output: 1
print(my_tuple[1:3]) # Output: (2, 3)
Tuple Operations
Tuples support fewer operations than lists, since they are immutable. However, you can still use the index()
and count()
methods to find the index of an element or count the occurrences of an element in the tuple:
my_tuple = (1, 2, 3, 4, 5)
print(my_tuple.index(3)) # Output: 2
print(my_tuple.count(2)) # Output: 1
Dictionaries
Dictionaries are data structures that store key-value pairs, where keys are unique strings and values can be of any data type. Dictionaries are denoted by curly braces {}
and are mutable.
Creating a Dictionary
To create a dictionary, you can use the dict()
function or simply enclose the key-value pairs in curly braces:
my_dict = dict({'name': 'John', 'age': 30})
or
my_dict = {'name': 'John', 'age': 30}
Accessing Dictionary Elements
Dictionary elements can be accessed using the key:
my_dict = {'name': 'John', 'age': 30}
print(my_dict['name']) # Output: John
Dictionary Operations
Dictionaries support various operations, including:
- Update: updates the value of an existing key or adds a new key-value pair
- Get: retrieves the value of a key
- Keys: returns a list of all keys in the dictionary
- Values: returns a list of all values in the dictionary
- Items: returns a list of all key-value pairs in the dictionary
- Pop: removes a key-value pair from the dictionary
my_dict = {'name': 'John', 'age': 30}
my_dict.update({'city': 'New York'}) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
print(my_dict.get('name')) # Output: John
print(my_dict.keys()) # Output: ['name', 'age', 'city']
print(my_dict.values()) # Output: ['John', 30, 'New York']
print(my_dict.items()) # Output: [('name', 'John'), ('age', 30), ('city', 'New York')]
my_dict.pop('city') # Output: {'name': 'John', 'age': 30}
Sets
Sets are unordered collections of unique elements, often used to remove duplicates from a list or to perform set operations. Sets are denoted by curly braces {}
and are mutable.
Creating a Set
To create a set, you can use the set()
function or simply enclose the elements in curly braces:
my_set = set([1, 2, 3, 4, 5])
or
my_set = {1, 2, 3, 4, 5}
Set Operations
Sets support various operations, including:
- Union: returns a new set with all elements from both sets
- Intersection: returns a new set with elements common to both sets
- Difference: returns a new set with elements in the first set but not in the second set
- Symmetric Difference: returns a new set with elements in either set but not in both
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7, 8}
print(set1.union(set2)) # Output: {1, 2, 3, 4, 5, 6, 7, 8}
print(set1.intersection(set2)) # Output: {4, 5}
print(set1.difference(set2)) # Output: {1, 2, 3}
print(set1.symmetric_difference(set2)) # Output: {1, 2, 3, 6, 7, 8}
Conclusion
In this blog post, we explored the different data structures in Python, including lists, tuples, dictionaries, and sets. Understanding these data structures is crucial to write efficient and effective code in Python. By mastering these data structures, you can improve your programming skills and tackle complex problems with ease.
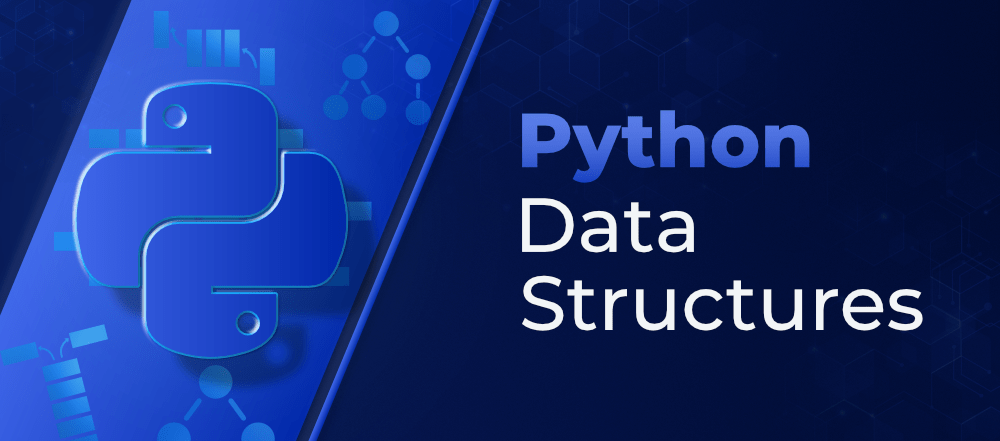
Remember, practice makes perfect. Start experimenting with these data structures today and see how you can apply them to real-world problems. Happy coding!