- Published on
Mastering Java Data Structures for Efficient Programming
- Authors
- Name
- Adil ABBADI
Introduction
Java is a popular programming language used for developing a wide range of applications, from mobile apps to enterprise software. At the heart of any programming language lies its data structures, which determine the efficiency and scalability of the code. In this blog post, we will delve into the world of Java data structures, exploring the most common ones, their implementation, and real-world use cases.
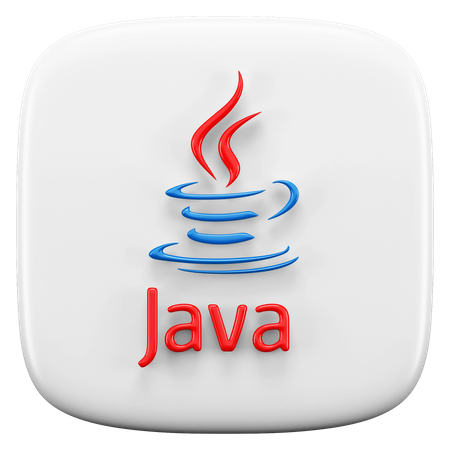
- Arrays in Java
- LinkedList in Java
- Stack in Java
- Queue in Java
- HashSet in Java
- TreeSet in Java
- HashMap in Java
- Conclusion
- Ready to Master Java Data Structures?
Arrays in Java
Arrays are the most basic data structure in Java, used to store a collection of elements of the same data type. They are homogeneous, which means all elements must be of the same type.
int[] myArray = {1, 2, 3, 4, 5};
LinkedList in Java
A LinkedList is a dynamic data structure that consists of a sequence of nodes, each pointing to the next node. It's useful for scenarios where frequent insertions or deletions occur.
import java.util.LinkedList;
LinkedList<String> myLinkedList = new LinkedList<>();
myLinkedList.add("Apple");
myLinkedList.add("Banana");
myLinkedList.add("Cherry");
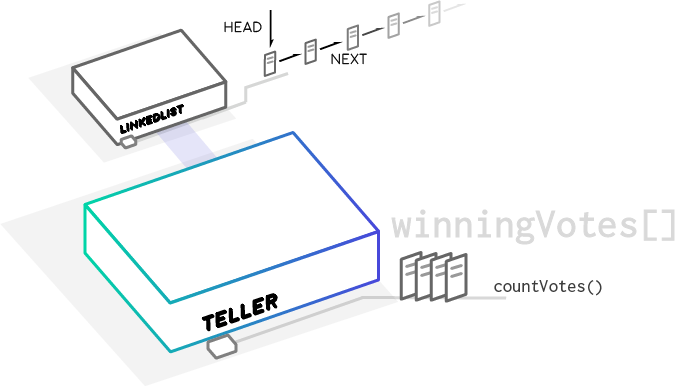
Stack in Java
A Stack is a Last-In-First-Out (LIFO) data structure, where elements are added and removed from the top of the stack.
import java.util.Stack;
Stack<Integer> myStack = new Stack<>();
myStack.push(1);
myStack.push(2);
myStack.push(3);
Queue in Java
A Queue is a First-In-First-Out (FIFO) data structure, where elements are added to the end and removed from the front of the queue.
import java.util.Queue;
import java.util.LinkedList;
Queue<String> myQueue = new LinkedList<>();
myQueue.add("Apple");
myQueue.add("Banana");
myQueue.add("Cherry");
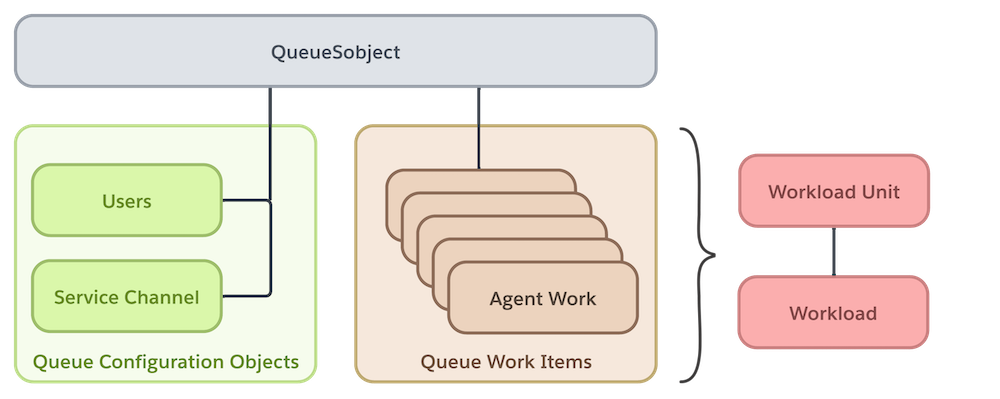
HashSet in Java
A HashSet is a collection of unique elements, without any specific order. It's useful for scenarios where fast lookup and insertion are required.
import java.util.HashSet;
HashSet<String> myHashSet = new HashSet<>();
myHashSet.add("Apple");
myHashSet.add("Banana");
myHashSet.add("Cherry");
TreeSet in Java
A TreeSet is a sorted collection of unique elements, which maintains a natural ordering of its elements.
import java.util.TreeSet;
TreeSet<String> myTreeSet = new TreeSet<>();
myTreeSet.add("Apple");
myTreeSet.add("Banana");
myTreeSet.add("Cherry");
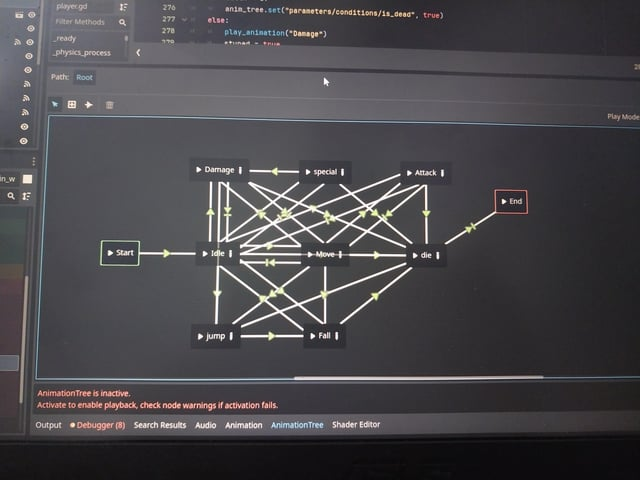
HashMap in Java
A HashMap is a collection of key-value pairs, where each key is unique and maps to a specific value.
import java.util.HashMap;
HashMap<String, Integer> myHashMap = new HashMap<>();
myHashMap.put("Apple", 1);
myHashMap.put("Banana", 2);
myHashMap.put("Cherry", 3);
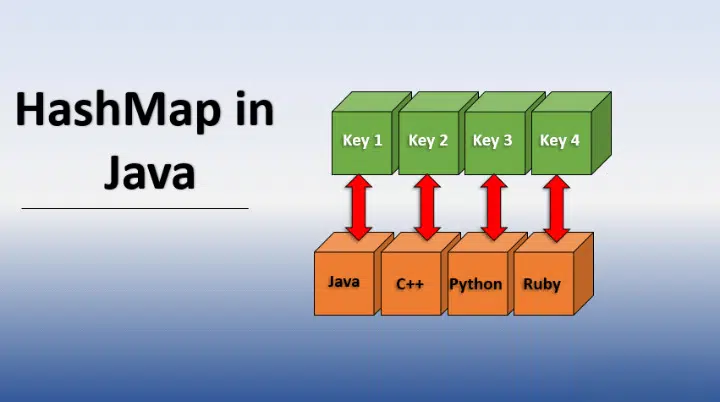
Conclusion
In this blog post, we explored the world of Java data structures, covering arrays, LinkedList, Stack, Queue, HashSet, TreeSet, and HashMap. Each data structure has its own strengths and weaknesses, and choosing the right one depends on the specific requirements of your application. By mastering these data structures, you can write efficient and scalable code, improving the overall performance of your Java applications.
Ready to Master Java Data Structures?
Start improving your Java skills today and become proficient in using the right data structures for your projects.