- Published on
Mastering Python List Comprehensions A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
In Python, list comprehensions are a powerful tool for creating lists in a concise and expressive way. They provide a concise syntax for creating lists from scratch, and are often used to simplify code and improve performance. In this blog post, we'll explore the basics, advanced techniques, and best practices of using Python list comprehensions to write efficient and readable code.
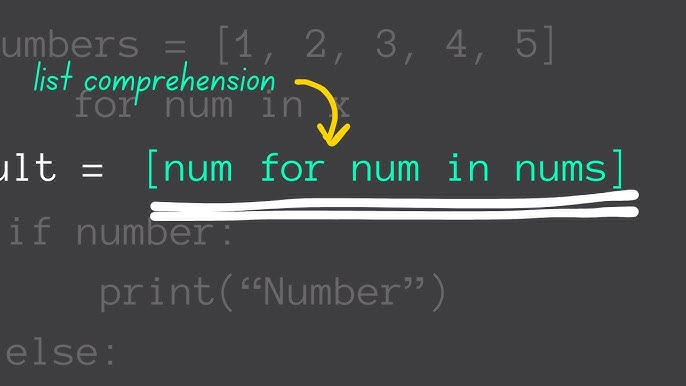
- Basic List Comprehensions
- Advanced List Comprehensions
- Nested List Comprehensions
- List Comprehensions with Dictionary
- Best Practices
- Conclusion
- Ready to Master List Comprehensions?
Basic List Comprehensions
A basic list comprehension consists of three parts: the input, the operation, and the output. The input is the iterable that you want to process, the operation is the expression that is applied to each element of the input, and the output is the resulting list.
Here is a simple example of a list comprehension:
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
print(squares) # [1, 4, 9, 16, 25]
In this example, the input is the numbers
list, the operation is squaring each element, and the output is a new list containing the squares of the original elements.
Advanced List Comprehensions
List comprehensions can also be used with conditional statements to filter the input elements. This is done using the if
keyword.
Here is an example of a list comprehension with a conditional statement:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = [x for x in numbers if x % 2 == 0]
print(even_numbers) # [2, 4, 6, 8, 10]
In this example, the input is the numbers
list, the operation is selecting only the elements that are even, and the output is a new list containing only the even numbers.
Nested List Comprehensions
List comprehensions can also be nested to create complex data structures. This is done by placing one list comprehension inside another.
Here is an example of a nested list comprehension:
numbers = [1, 2, 3]
letters = ['a', 'b', 'c']
combinations = [(x, y) for x in numbers for y in letters]
print(combinations) # [(1, 'a'), (1, 'b'), (1, 'c'), (2, 'a'), (2, 'b'), (2, 'c'), (3, 'a'), (3, 'b'), (3, 'c')]
In this example, the input is two lists numbers
and letters
, the operation is creating a tuple for each combination of elements from both lists, and the output is a new list containing all possible combinations.
List Comprehensions with Dictionary
List comprehensions can also be used to create dictionaries. This is done by using the dict
constructor and a list comprehension.
Here is an example of a list comprehension with a dictionary:
numbers = [1, 2, 3]
squares_dict = {x: x**2 for x in numbers}
print(squares_dict) # {1: 1, 2: 4, 3: 9}
In this example, the input is the numbers
list, the operation is creating a key-value pair for each element, and the output is a new dictionary containing the squares of the original elements.
Best Practices
Here are some best practices to keep in mind when using list comprehensions:
- Use them sparingly: List comprehensions can make your code more concise, but they can also make it less readable if overused.
- Use descriptive variable names: Use descriptive variable names to make it clear what the list comprehension is doing.
- Avoid complex logic: Avoid putting complex logic inside a list comprehension. Instead, break it down into smaller, simpler functions.
- Test them thoroughly: Test your list comprehensions thoroughly to ensure they are working as expected.
Conclusion
In this blog post, we explored the basics, advanced techniques, and best practices of using Python list comprehensions to write efficient and readable code. By mastering list comprehensions, you can write more concise and expressive code, and take your Python skills to the next level.
Ready to Master List Comprehensions?
Start improving your coding skills today and become proficient in using Python list comprehensions to write efficient and readable code.