- Published on
Mastering Data Structures with C++ A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
In computer science, data structures play a vital role in efficiently storing, organizing, and retrieving data. C++ is a powerful programming language that provides low-level memory management and performance, making it an ideal choice for implementing data structures. In this comprehensive guide, we will explore the world of data structures using C++ and learn how to effectively implement and manipulate them.
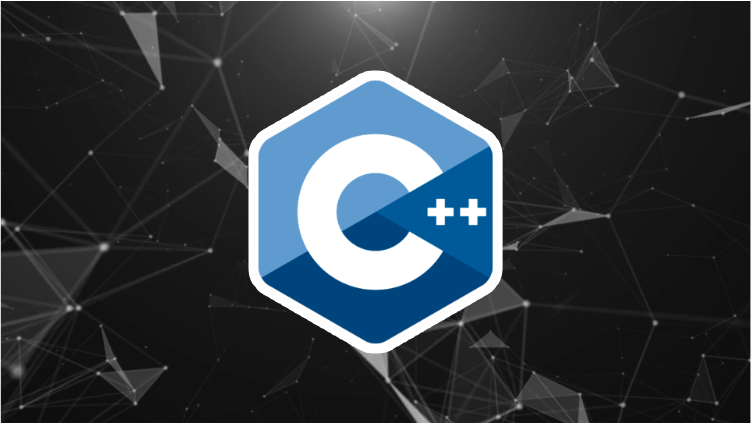
- Why C++ for Data Structures?
- Basic Data Structures in C++
- Advanced Data Structures in C++
- Implementing Data Structures in C++
- Real-World Applications of Data Structures
- Conclusion
- Ready to Master Data Structures in C++?
Why C++ for Data Structures?
C++ is a popular choice for implementing data structures due to its:
- Performance: C++ provides low-level memory management, allowing for efficient memory allocation and deallocation.
- Control: C++ offers direct access to hardware resources, enabling precise control over data structure implementation.
- Flexibility: C++ supports both object-oriented and procedural programming paradigms, making it suitable for various data structure implementations.
Basic Data Structures in C++
Before diving into more complex data structures, let's start with the basics:
Arrays
Arrays are a fundamental data structure in C++. They are used to store a collection of elements of the same data type.
int arr[5] = {1, 2, 3, 4, 5};
Linked Lists
Linked lists are a dynamic data structure that consists of nodes, each containing a value and a reference to the next node.
struct Node {
int data;
Node* next;
};
Node* head = new Node;
head->data = 1;
head->next = new Node;
head->next->data = 2;
Stacks
Stacks are a Last-In-First-Out (LIFO) data structure, commonly used for evaluating postfix expressions and parsing.
class Stack {
private:
int arr[5];
int top;
public:
Stack() : top(-1) {}
void push(int element) {
arr[++top] = element;
}
int pop() {
return arr[top--];
}
};
Advanced Data Structures in C++
Now that we've covered the basics, let's move on to more complex data structures:
Binary Trees
Binary trees are a hierarchical data structure, commonly used for database indexing and file systems.
struct Node {
int data;
Node* left;
Node* right;
};
Node* root = new Node;
root->data = 1;
root->left = new Node;
root->left->data = 2;
root->right = new Node;
root->right->data = 3;
Hash Tables
Hash tables are a data structure that stores key-value pairs using a hash function.
class HashTable {
private:
int capacity;
int* keys;
int* values;
public:
HashTable(int capacity) : capacity(capacity) {
keys = new int[capacity];
values = new int[capacity];
}
void insert(int key, int value) {
int index = hash(key);
keys[index] = key;
values[index] = value;
}
int get(int key) {
int index = hash(key);
return values[index];
}
int hash(int key) {
return key % capacity;
}
};
Graphs
Graphs are a non-linear data structure, commonly used for social networks and web graphs.
class Graph {
private:
int vertices;
int** adjacencyMatrix;
public:
Graph(int vertices) : vertices(vertices) {
adjacencyMatrix = new int*[vertices];
for (int i = 0; i < vertices; i++) {
adjacencyMatrix[i] = new int[vertices];
}
}
void addEdge(int src, int dest) {
adjacencyMatrix[src][dest] = 1;
}
void printGraph() {
for (int i = 0; i < vertices; i++) {
for (int j = 0; j < vertices; j++) {
std::cout << adjacencyMatrix[i][j] << " ";
}
std::cout << std::endl;
}
}
};
Implementing Data Structures in C++
When implementing data structures in C++, keep the following best practices in mind:
- Use meaningful variable names: Choose variable names that accurately reflect their purpose.
- Follow the SOLID principles: Ensure your code adheres to the Single Responsibility Principle (SRP), Open-Closed Principle (OCP), Liskov Substitution Principle (LSP), Interface Segregation Principle (ISP), and Dependency Inversion Principle (DIP).
- Use smart pointers: Utilize smart pointers like
unique_ptr
andshared_ptr
to manage memory efficiently.
Real-World Applications of Data Structures
Data structures have numerous real-world applications, including:
- Database Systems: Data structures like B-Trees and Hash Tables are used in database systems for efficient data retrieval.
- Social Networks: Graphs are used to represent social networks and analyze relationships between users.
- File Systems: Data structures like Trees and Hash Tables are used in file systems for efficient file organization and retrieval.
Conclusion
In this comprehensive guide, we explored the world of data structures using C++. By mastering the implementation and manipulation of data structures, you can write efficient and effective code for a wide range of applications.
Ready to Master Data Structures in C++?
Start improving your skills today and become proficient in implementing and manipulating data structures in C++.

Remember to practice regularly and work on real-world projects to reinforce your understanding of data structures in C++.