- Published on
Mastering Data Analysis with Pandas
- Authors
- Name
- Adil ABBADI
Introduction
In the world of data analysis, Pandas has become an essential tool for data scientists and analysts. With its powerful data structures and efficient data manipulation capabilities, Pandas has revolutionized the way we work with data. In this blog post, we'll explore the ins and outs of using Pandas for data analysis, covering topics such as importing and cleaning data, data visualization, and data transformation.
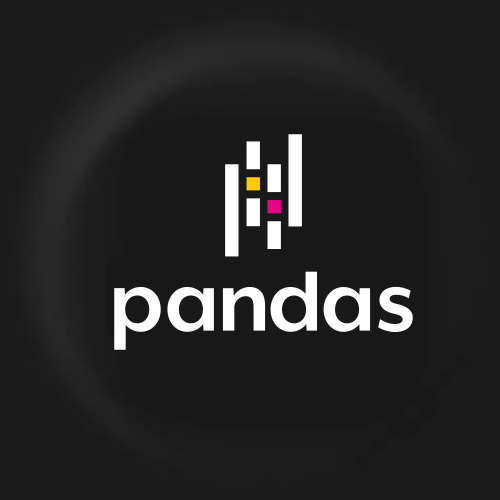
- Importing and Cleaning Data with Pandas
- Data Visualization with Pandas
- Data Transformation with Pandas
- Practical Example of Data Analysis with Pandas
- Conclusion
- Ready to Master Data Analysis with Pandas?
Importing and Cleaning Data with Pandas
The first step in any data analysis project is importing and cleaning the data. Pandas provides several ways to import data, including from CSV, Excel, and SQL databases.
Importing Data from CSV Files
To import data from a CSV file, you can use the read_csv()
function:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.head())
This code imports the data from the data.csv
file and prints the first few rows of the data using the head()
function.
Handling Missing Values
Real-world data often contains missing values, which can cause problems during analysis. Pandas provides several ways to handle missing values, including:
dropna()
: drops rows or columns with missing valuesfillna()
: fills missing values with a specific value or methodisna()
: checks for missing values
Here's an example of using dropna()
to drop rows with missing values:
df = df.dropna()
print(df.head())
Data Cleaning and Preprocessing
Data cleaning and preprocessing are essential steps in data analysis. Pandas provides several functions for cleaning and preprocessing data, including:
strip()
: removes whitespace from stringslower()
: converts strings to lowercaseupper()
: converts strings to uppercaseastype()
: converts data types
Here's an example of using strip()
to remove whitespace from strings:
df['column_name'] = df['column_name'].str.strip()
print(df.head())
Data Visualization with Pandas
Data visualization is an essential part of data analysis, as it helps us understand and communicate insights from the data. Pandas integrates well with popular data visualization libraries like Matplotlib and Seaborn.
Plotting with Matplotlib
To plot data with Matplotlib, you can use the plot()
function:
import matplotlib.pyplot as plt
df.plot(kind='bar')
plt.show()
This code plots the data as a bar chart using Matplotlib.
Plotting with Seaborn
To plot data with Seaborn, you can use the sns
library:
import seaborn as sns
sns.set_style('whitegrid')
sns.barplot(x='column_name', y='column_name', data=df)
plt.show()
This code plots the data as a bar chart using Seaborn.
Data Transformation with Pandas
Data transformation is an essential part of data analysis, as it helps us prepare the data for modeling and analysis. Pandas provides several functions for data transformation, including:
groupby()
: groups data by one or more columnspivot_table()
: creates a pivot table from the datamelt()
: melts the data from wide format to long format
Here's an example of using groupby()
to group data by a column:
df.groupby('column_name').agg({'column_name': 'sum'})
This code groups the data by the column_name
column and calculates the sum of the column_name
column.
Practical Example of Data Analysis with Pandas
Here's a practical example of using Pandas for data analysis:
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
# import data from a CSV file
df = pd.read_csv('data.csv')
# handle missing values
df = df.dropna()
# clean and preprocess data
df['column_name'] = df['column_name'].str.strip()
# visualize data
df.plot(kind='bar')
plt.show()
# transform data
df_grouped = df.groupby('column_name').agg({'column_name': 'sum'})
# visualize transformed data
sns.barplot(x='column_name', y='column_name', data=df_grouped)
plt.show()
This code imports data from a CSV file, handles missing values, cleans and preprocesses the data, visualizes the data, transforms the data, and visualizes the transformed data.
Conclusion
In this blog post, we explored the power of Pandas for data analysis, covering topics such as importing and cleaning data, data visualization, and data transformation. By mastering Pandas, you can efficiently and effectively analyze and visualize data to gain insights and make informed decisions.
Ready to Master Data Analysis with Pandas?
Start improving your data analysis skills today and become proficient in using Pandas for robust data manipulation and visualization.