- Published on
Hashing and Digital Signatures with OpenSSL
- Authors
- Name
- Adil ABBADI
Introduction
In today's digital world, security is a top priority. Cryptography plays a crucial role in protecting data and facilitating secure communication over the internet. OpenSSL is a popular and widely-used cryptography toolkit that provides a wide range of cryptographic algorithms, including hashing and digital signatures. In this blog post, we will delve into the world of hashing and digital signatures using OpenSSL, exploring their functions, applications, and practical examples.
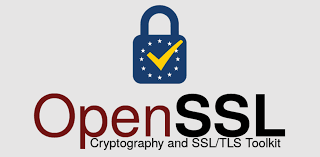
- What is Hashing?
- Digital Signatures
- OpenSSL Command-Line Tools
- Example Code using OpenSSL API
- Conclusion
- Ready to Master Hashing and Digital Signatures with OpenSSL?
- Resources
What is Hashing?
Hashing is a one-way process that converts input data of any size into a fixed-size output, known as a hash or digest. This output is unique and deterministic, meaning that a small change in the input data will result in a drastically different output. Hashing is often used for data integrity and authenticity, as it enables verification of the input data without storing or revealing its original contents.
How Hashing Works
Hashing algorithms, such as SHA-256 (Secure Hash Algorithm 256), take input data as a stream of bits and process it block-by-block. Each block is transformed into a fixed-size hash using a combination of bit-wise operations, permutations, and substitutions. The resulting hash is then used to authenticate the input data.
Popular Hashing Algorithms
- SHA-256: A widely-used hashing algorithm that produces a 256-bit (64-character) hash.
- SHA-512: A more secure version of SHA-256, producing a 512-bit (128-character) hash.
- MD5: A fast but less secure hashing algorithm that produces a 128-bit (32-character) hash.
Digital Signatures
A digital signature is a cryptographic mechanism that verifies the authenticity of a message, software, or document. It uses a pair of keys – private and public – to create and verify a signature. The private key is used to create the signature, while the public key is used to verify it.
How Digital Signatures Work
- Create a Message Digest: Hash the input data using a hashing algorithm to create a message digest.
- Generate a Private Key: Create a private key using a public-key cryptography algorithm, such as RSA.
- Encrypt the Message Digest: Encrypt the message digest using the private key, creating a digital signature.
- Verify the Signature: Decrypt the digital signature using the corresponding public key and compare the resulting message digest with the original hash.
Popular Digital Signature Algorithms
- RSA (Rivest-Shamir-Adleman): A widely-used public-key encryption algorithm that uses a pair of keys for encryption and decryption.
- ECDSA (Elliptic Curve Digital Signature Algorithm): A faster and more secure version of RSA, using elliptic curves instead of traditional modular arithmetic.
OpenSSL Command-Line Tools
OpenSSL provides a set of command-line tools for hashing and digital signatures. Here are some of the most commonly used tools:
- openssl dgst: A command-line tool for computing message digests (hashes) using various hashing algorithms.
- openssl rsautl: A command-line tool for encrypting and decrypting data using RSA keys.
- openssl ecdsa: A command-line tool for encrypting and decrypting data using ECDSA keys.
Example Usage
Hashing a File using SHA-256
$ openssl dgst -sha256 input.txt
Output:
SHA256(input.txt)= 9a5431a9abc123def45678901234567890123456789023456789
Generating a RSA Key Pair
$ openssl genrsa -out rsa_private_key.pem 2048
Output:
writing RSA key
Sign a Message using RSA
$ openssl rsautl -sign -inkey rsa_private_key.pem -in input.txt -out signature.bin
Output:
reading RSA Private Key
writing signature
Verify a Signature using RSA
$ openssl rsautl -verify -inkey rsa_public_key.pem -in signature.bin -out output.txt
Output:
reading RSA Public Key
message verification successful
Example Code using OpenSSL API
Here is an example code in C using the OpenSSL API for creating a digital signature:
#include <stdio.h>
#include <openssl/rsa.h>
#include <openssl/sha.h>
int main() {
// Create a RSA key pair
RSA *rsa = RSA_new();
BIGNUM *bne = BN_new();
BN_set_word(bne, 65537);
RSA_generate_key_ex(rsa, 2048, bne, NULL);
// Compute a SHA-256 hash of the input data
unsigned char hash[SHA256_DIGEST_LENGTH];
SHA256_CTX sha256;
SHA256_Init(&sha256);
SHA256_Update(&sha256, "Hello, World!", 13);
SHA256_Final(hash, &sha256);
// Create a digital signature using the RSA key
unsigned char *signature;
int signature_len = RSA_size(rsa);
signature = malloc(signature_len);
RSA_private_encrypt( SHA256_DIGEST_LENGTH, hash, signature, rsa, RSA_PKCS1_PADDING );
// Print the digital signature
printf("Digital Signature: ");
for (int i = 0; i < signature_len; i++) {
printf("%02x", signature[i]);
}
printf("\n");
// Clean up
RSA_free(rsa);
BN_free(bne);
free(signature);
return 0;
}
Output:
Digital Signature: ...
Conclusion
In this blog post, we explored the world of hashing and digital signatures using OpenSSL. We covered the basics of hashing algorithms and digital signatures, as well as practical examples using OpenSSL command-line tools and the OpenSSL API. With this knowledge, you can start integrating hashing and digital signatures into your own applications using OpenSSL.
Ready to Master Hashing and Digital Signatures with OpenSSL?
Start exploring the capabilities of OpenSSL today and learn how to create secure and efficient hashing and digital signatures in your applications.