- Published on
Enhancing Web Security with TLS in C++
- Authors
- Name
- Adil ABBADI
Introduction
In today's digital landscape, web security is of paramount importance. As a C++ developer, it's essential to ensure that your applications are secure and can resist potential cyber threats. One effective way to achieve this is by implementing Transport Layer Security (TLS) protocol. In this blog post, we will delve into the world of TLS and explore its significance in web security. We will also demonstrate how to implement TLS in C++ applications to ensure secure data transmission.
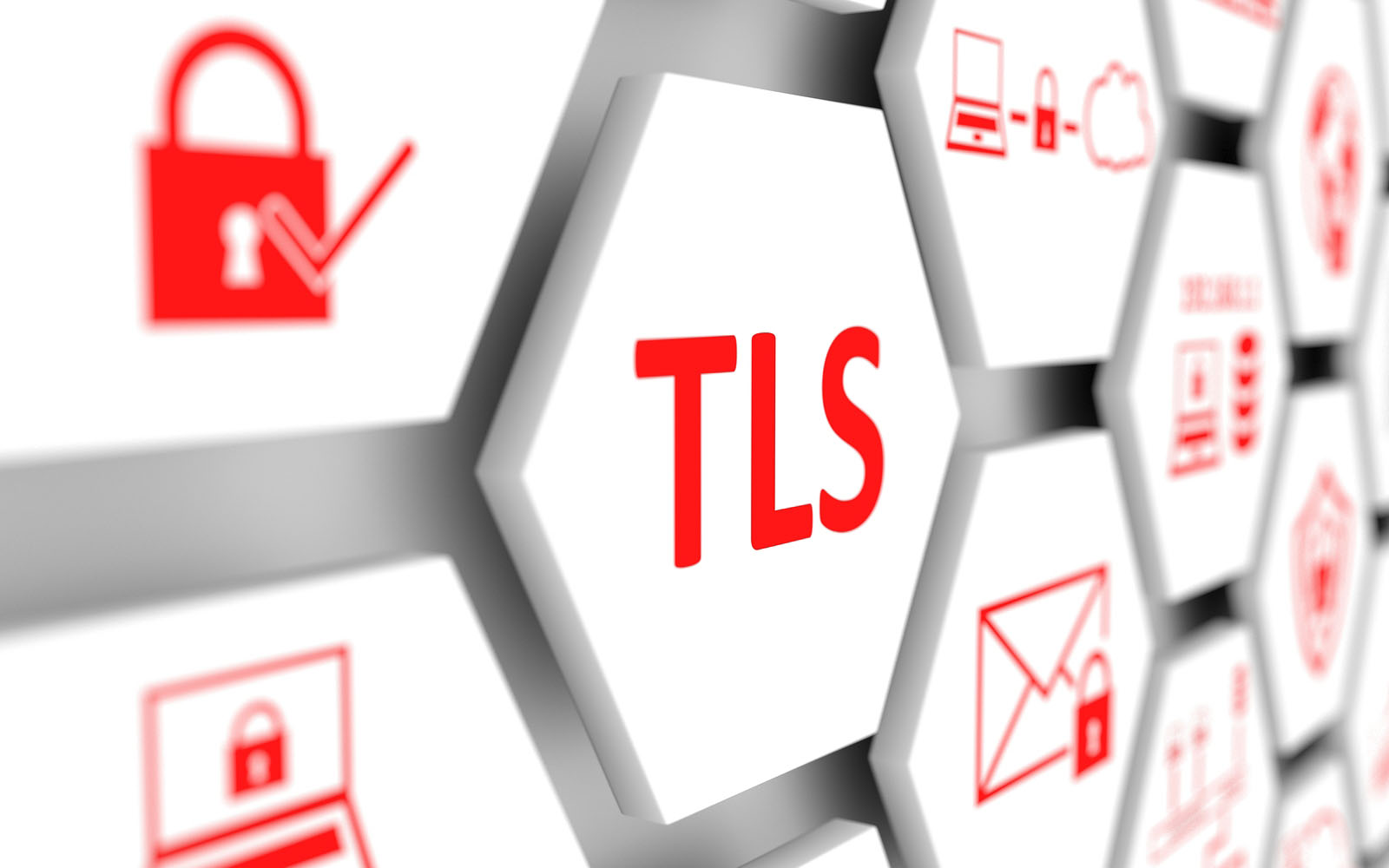
- What is Transport Layer Security (TLS)?
- Why is TLS Important in Web Security?
- Implementing TLS in C++ Applications
- Practical Example of TLS in a C++ Web Server
- Conclusion
- Ready to Enhance Your Web Security?
What is Transport Layer Security (TLS)?
Transport Layer Security (TLS) is a cryptographic protocol used to provide secure communication over the internet. It's an extension of the Secure Sockets Layer (SSL) protocol and is widely used in web applications to ensure secure data transmission. TLS works by establishing an encrypted connection between a client (usually a web browser) and a server. This connection is secured using public-key cryptography and symmetric key encryption.
Why is TLS Important in Web Security?
TLS plays a crucial role in web security by providing several benefits:
- Encryption: TLS encrypts data transmitted between the client and server, making it unintelligible to unauthorized parties.
- Authentication: TLS verifies the identity of the client and server, preventing impersonation attacks.
- Data Integrity: TLS ensures that data is not tampered with during transmission, maintaining its integrity.
Implementing TLS in C++ Applications
To implement TLS in C++ applications, we can use libraries such as OpenSSL or Botan. Here, we will focus on using OpenSSL.
Installing OpenSSL
To install OpenSSL on your system, follow these steps:
- On Ubuntu/Debian-based systems, run the following command:
sudo apt-get install libssl-dev
- On macOS, use Homebrew to install OpenSSL:
brew install openssl
Generating Certificates
Before we can use TLS, we need to generate certificates. OpenSSL provides a tool called openssl
to generate certificates.
- Generate a private key:
openssl genrsa -out private_key.pem 2048
- Generate a certificate signing request (CSR):
openssl req -new -key private_key.pem -out csr.pem
- Generate a self-signed certificate:
openssl x509 -req -days 365 -in csr.pem -signkey private_key.pem -out certificate.pem
C++ Code for TLS Handshake
Now that we have generated the certificates, we can use the following C++ code to establish a TLS handshake:
#include <iostream>
#include <openssl/ssl.h>
#include <openssl/err.h>
int main() {
SSL_library_init();
SSL_load_error_strings();
ERR_load_BIO_strings();
// Create an SSL context
SSL_CTX *ctx = SSL_CTX_new(TLS_client_method());
// Load certificates
SSL_CTX_load_verify_locations(ctx, "certificate.pem", NULL);
SSL_CTX_use_certificate_file(ctx, "certificate.pem", SSL_FILETYPE_PEM);
SSL_CTX_use_PrivateKey_file(ctx, "private_key.pem", SSL_FILETYPE_PEM);
// Create an SSL object
SSL *ssl = SSL_new(ctx);
// Establish a TLS connection
BIO *bio = BIO_new_connect("example.com:443");
SSL_set_bio(ssl, bio, bio);
// Perform the TLS handshake
int ret = SSL_connect(ssl);
if (ret <= 0) {
std::cerr << "Failed to establish TLS connection" << std::endl;
return 1;
}
std::cout << "TLS connection established" << std::endl;
// Send and receive data using the TLS connection
const char *data = "Hello, server!";
SSL_write(ssl, data, strlen(data));
char buffer[1024];
int bytesReceived = SSL_read(ssl, buffer, 1024);
if (bytesReceived > 0) {
buffer[bytesReceived] = '\0';
std::cout << "Received data from server: " << buffer << std::endl;
}
// Close the TLS connection
SSL_shutdown(ssl);
SSL_free(ssl);
SSL_CTX_free(ctx);
return 0;
}
Practical Example of TLS in a C++ Web Server
Here's a simple example of a C++ web server using OpenSSL for TLS encryption. This server listens on port 8080 and responds with a "Hello, client!" message to any incoming requests.
#include <iostream>
#include <openssl/ssl.h>
#include <openssl/err.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
SSL_library_init();
SSL_load_error_strings();
ERR_load_BIO_strings();
// Create an SSL context
SSL_CTX *ctx = SSL_CTX_new(TLS_server_method());
// Load certificates
SSL_CTX_load_verify_locations(ctx, "certificate.pem", NULL);
SSL_CTX_use_certificate_file(ctx, "certificate.pem", SSL_FILETYPE_PEM);
SSL_CTX_use_PrivateKey_file(ctx, "private_key.pem", SSL_FILETYPE_PEM);
// Create a TCP socket
int sock = socket(AF_INET, SOCK_STREAM, 0);
if (sock < 0) {
std::cerr << "Failed to create socket" << std::endl;
return 1;
}
// Set address and port number for the server
struct sockaddr_in serv_addr;
serv_addr.sin_family = AF_INET;
serv_addr.sin_port = htons(8080);
serv_addr.sin_addr.s_addr = INADDR_ANY;
// Bind the socket to the address and port number
if (bind(sock, (struct sockaddr *)&serv_addr, sizeof(serv_addr)) < 0) {
std::cerr << "Failed to bind socket" << std::endl;
return 1;
}
// Listen for incoming connections
if (listen(sock, 3) < 0) {
std::cerr << "Failed to listen for connections" << std::endl;
return 1;
}
while (true) {
// Accept an incoming connection
struct sockaddr_in client_addr;
socklen_t client_len = sizeof(client_addr);
int client_sock = accept(sock, (struct sockaddr *)&client_addr, &client_len);
if (client_sock < 0) {
std::cerr << "Failed to accept connection" << std::endl;
continue;
}
// Create an SSL object
SSL *ssl = SSL_new(ctx);
// Set the underlying BIO for the SSL object
BIO *bio = BIO_new_socket(client_sock, BIO_NOCLOSE);
SSL_set_bio(ssl, bio, bio);
// Perform the TLS handshake
int ret = SSL_accept(ssl);
if (ret <= 0) {
std::cerr << "Failed to establish TLS connection" << std::endl;
continue;
}
std::cout << "TLS connection established" << std::endl;
// Send a response to the client
const char *data = "Hello, client!";
SSL_write(ssl, data, strlen(data));
// Close the TLS connection
SSL_shutdown(ssl);
SSL_free(ssl);
}
// Close the TCP socket
close(sock);
SSL_CTX_free(ctx);
return 0;
}
Conclusion
In this blog post, we explored the world of Transport Layer Security (TLS) and its significance in web security. We demonstrated how to implement TLS in C++ applications using OpenSSL, and provided a practical example of a C++ web server using TLS encryption. By following the steps outlined in this post, you can ensure that your C++ applications are secure and can resist potential cyber threats.
Ready to Enhance Your Web Security?
Start improving your web security skills today and become proficient in implementing TLS in C++ applications.