- Published on
TensorFlow for Deep Learning A Comprehensive Guide
- Authors
- Name
- Adil ABBADI
Introduction
Deep learning has revolutionized the field of artificial intelligence, enabling machines to learn and improve on their own by automatically extracting features from data. TensorFlow, an open-source library developed by Google, is one of the most popular frameworks for building and training deep learning models. In this comprehensive guide, we will explore the world of TensorFlow for deep learning, covering its architecture, basic components, and advanced features.
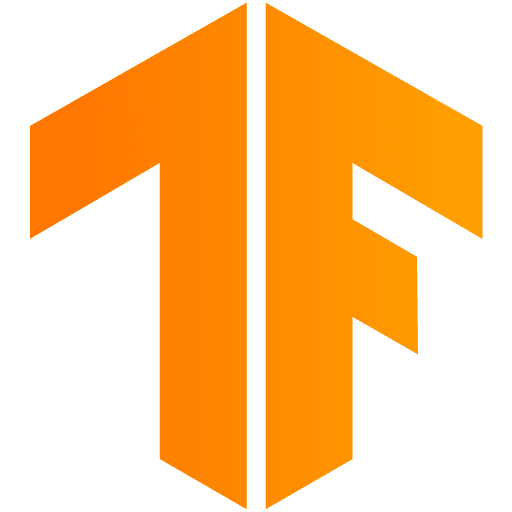
- What is TensorFlow?
- Basic Components of TensorFlow
- Building a Simple Neural Network with TensorFlow
- Advanced Features of TensorFlow
- Real-World Applications of TensorFlow
- Conclusion
- Ready to Start with TensorFlow?
What is TensorFlow?
TensorFlow is an open-source software library for numerical computation, particularly well-suited and fine-tuned for large-scale Machine Learning (ML) and Deep Learning (DL) tasks. Its primary use is in developing and training artificial neural networks, particularly deep neural networks.
TensorFlow allows developers to easily implement popular DL architectures such as Convolutional Neural Networks (CNNs), Recurrent Neural Networks (RNNs), and Autoencoders.
Basic Components of TensorFlow
TensorFlow's architecture is based on the following basic components:
Tensors
Tensors are the fundamental data structures in TensorFlow. A tensor is a multidimensional array of numerical values.
Nodes
Nodes are the computational units in a TensorFlow graph. They represent mathematical operations such as addition, multiplication, and convolution.
Graph
A graph is a collection of nodes and edges that define the computational flow of a TensorFlow model.
Sessions
A session is an environment in which the graph is executed.
Variables
Variables are used to store and update the values of tensors during training.
Building a Simple Neural Network with TensorFlow
Let's build a simple neural network using TensorFlow to classify handwritten digits using the MNIST dataset.
import tensorflow as tf
from tensorflow.keras.datasets import mnist
# Load MNIST dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
# Reshape and normalize the data
X_train = X_train.reshape(-1, 28, 28, 1).astype('float32') / 255
X_test = X_test.reshape(-1, 28, 28, 1).astype('float32') / 255
# Build the model
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# Train the model
model.fit(X_train, y_train, epochs=5, batch_size=128, validation_data=(X_test, y_test))
Advanced Features of TensorFlow
TensorFlow provides several advanced features that make it a powerful tool for deep learning:
Automatic Differentiation
TensorFlow provides automatic differentiation, which enables the computation of gradients of a loss function with respect to model parameters.
Distributed Training
TensorFlow allows distributed training, which enables the training of large models on multiple machines.
TensorFlow Estimators
TensorFlow Estimators provide a high-level interface for building and training machine learning models.
TensorFlow Serving
TensorFlow Serving is a system for deploying and managing machine learning models in production environments.
TensorFlow Lite
TensorFlow Lite is a lightweight version of TensorFlow for mobile and embedded devices.
TensorFlow.js
TensorFlow.js is a JavaScript version of TensorFlow for client-side machine learning.
Real-World Applications of TensorFlow
TensorFlow has numerous real-world applications, including:
Computer Vision
TensorFlow is widely used in computer vision applications such as object detection, segmentation, and image classification.
Natural Language Processing
TensorFlow is used in natural language processing applications such as language modeling, text classification, and machine translation.
Speech Recognition
TensorFlow is used in speech recognition applications such as voice assistants and speech-to-text systems.
Robotics
TensorFlow is used in robotics applications such as control systems and motion planning.
Conclusion
TensorFlow is a powerful tool for deep learning, providing an efficient and flexible way to build and train neural networks. With its advanced features and real-world applications, TensorFlow is an essential tool for anyone interested in machine learning and artificial intelligence.
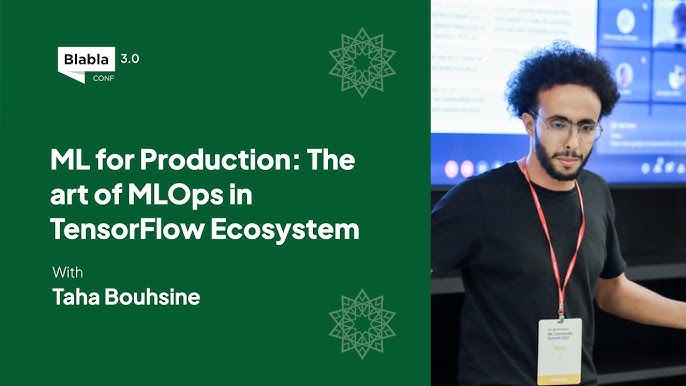
Ready to Start with TensorFlow?
Start building your deep learning models with TensorFlow today and explore the endless possibilities of artificial intelligence.
Happy learning!