- Published on
Mastering Spring Boot Start-up
- Authors
- Name
- Adil ABBADI
Introduction
Spring Boot is a popular Java framework for building web applications and microservices. One of the key features that make Spring Boot so appealing is its ability to simplify and streamline the application deployment process. In this blog post, we will delve into the world of Spring Boot start-up and explore the various strategies and best practices for efficiently and securely deploying your applications.
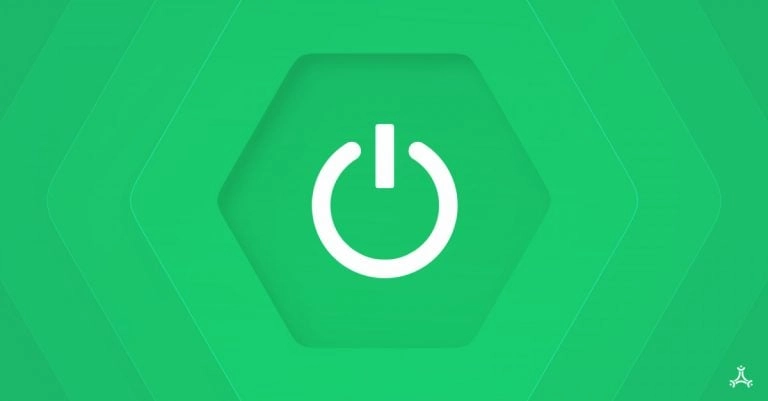
- Understanding the Spring Boot Life Cycle
- Spring Boot Start-up Strategies
- Best Practices for Spring Boot Start-up
- Conclusion
- Ready to Master Spring Boot Start-up?
Understanding the Spring Boot Life Cycle
Before diving into the various start-up strategies, it is essential to understand the Spring Boot life cycle. Spring Boot follows a well-defined life cycle that includes the following phases:
- Initialization: This phase involves initializing the Spring Boot application by loading the configuration files and creating the application context.
- Configuration: During this phase, the application context is populated with beans, and dependencies are injected.
- Bean Creation: In this phase, the beans are created, and their dependencies are resolved.
- Application Context Completion: The application context is completed, and the beans are fully initialized.
- Startup and Ready to Run: The application is started, and all the beans are fully initialized and ready to run.
Spring Boot Start-up Strategies
Now that we have an understanding of the Spring Boot life cycle, let's explore the various start-up strategies that can be employed to efficiently and securely deploy Spring Boot applications.
1. Disable Auto-Configuration
One of the key features of Spring Boot is its auto-configuration mechanism, which automatically enables certain features and beans based on the dependencies and properties present in the project. However, in some cases, auto-configuration may not be desirable, and disabling it can improve start-up time.
@SpringBootApplication
@EnableAutoConfiguration(exclude = {DataSourceAutoConfiguration.class})
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
2. Lazy Initialization
Lazy initialization is a feature that allows you to delay the initialization of beans until they are actually needed. This can significantly improve start-up time and reduce memory usage.
@Configuration
public class MyConfiguration {
@Lazy
@Bean
public MyBean myBean() {
// Create and return the bean
}
}
3. Use Executor Services
Using executor services can help improve the performance of your Spring Boot application by enabling asynchronous processing. This can be particularly useful when performing time-consuming operations.
@Configuration
public class MyConfiguration {
@Bean
public ExecutorService executorService() {
return Executors.newFixedThreadPool(5);
}
}
4. Optimize Database Configuration
Database configuration can be a significant bottleneck in the start-up process. Optimizing database configuration by using connection pools and caching can help improve performance.
@Configuration
public class MyDatabaseConfiguration {
@Bean
public DataSource dataSource() {
// Create and configure the DataSource
}
}
5. Leverage Cache
Caching can significantly improve the performance of your Spring Boot application by reducing the number of database queries and computations.
@Configuration
@EnableCaching
public class MyCacheConfiguration {
@Bean
public CacheManager cacheManager() {
return new SimpleCacheManager();
}
}
6. Use Externalized Configuration
Externalizing configuration can help improve start-up time by allowing you to configure your application at runtime.
# File: application.properties
server.port=8080
# File: application-startup.properties
startup.memory=-Xmx1024m
@Configuration
@PropertySource(name = "startup.config", value = "classpath:application-startup.properties")
public class MyStartUpConfiguration {
@Bean
public ServletApplicationInitializer applicationInitializer() {
return new MyApplicationInitializer();
}
}
Best Practices for Spring Boot Start-up
In addition to the various start-up strategies outlined above, there are several best practices that can be employed to ensure that your Spring Boot application starts up efficiently and securely:
- Use Spring Boot starters: Spring Boot starters can simplify the process of configuring and deploying your application.
- Minimize transitive dependencies: Transitive dependencies can significantly increase the size of your application jar.
- Use compiler optimizations: Compiler optimizations can help improve the performance of your application.
- Leverage profiling: Profiling can help identify performance bottlenecks in your application.
Conclusion
In this comprehensive guide to Spring Boot start-up, we explored various strategies and best practices for efficiently and securely deploying Spring Boot applications. By applying these strategies, you can significantly improve the performance and reliability of your Spring Boot applications.
Ready to Master Spring Boot Start-up?
Start optimizing your Spring Boot applications today and discover the benefits of efficient and secure deployment.